Quick Guide for Wiremock on Docker for Efficient Development
April 21, 2023 • 3 minutes • 543 words
As a developer working with microservices architecture, I know firsthand how challenging it can be to deal with dependencies on other services within the cluster or external APIs. It can sometimes slow down the development process and make debugging or testing a real headache.
This post is all about how I implemented a solution that made my development process a whole lot easier. Wiremock is a powerful and open-source mocking tool that can simulate external service behavior and allows you to create mock servers for more efficient testing and development.
How to set up a Wiremock server on Docker?
I’ll be using an example with one of my own favorite topics - WWE Wrestling!
The server will be called wwe-server
and we’re going to call api/v1/hall-of-fame
endpoint to get a list of hall of famers wrestlers.
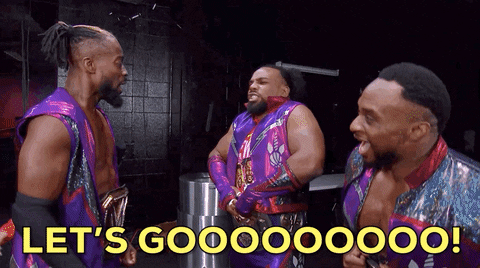
1 - Install Wiremock package
npx wiremock
2 - Add Wiremock Docker image
In your /docker-compose.yml
wiremock-wwe-server:
image: wiremock/wiremock:2.32.0
ports:
- 3001:8080
volumes:
- ./wiremock/wwe-server:/home/wiremock
3 - Create mock response files
Create a file wiremock/wwe-server/__files/hall-of-famers.json
[
{
"id": 1,
"stage_name": "Stone Cold Steve Austin",
"full_name": "Steve Austin",
"category": "Individual",
"createdAt": "2009-01-01T00:32:28.996Z",
"updatedAt": "2009-01-01T00:32:28.996Z",
},
{
"id": 2,
"stage_name": "Eddie Guerrero",
"full_name": "Eduardo Gory Guerrero Llanes",
"category": "Individual",
"createdAt": "2009-01-01T00:32:28.996Z",
"updatedAt": "2009-01-01T00:32:28.996Z",
},
{
"id": 3,
"stage_name": "The Undertaker",
"full_name": "Mark William Calaway",
"category": "Individual",
"createdAt": "2022-01-01T00:32:28.996Z",
"updatedAt": "2022-01-01T00:32:28.996Z",
}
]
4 - Map API calls
Create a file wiremock/wwe-server/mappings/hall-of-famers.json
{
"request": {
"method": "GET",
"urlPath": "/api/v1/hall-of-fame"
},
"response": {
"status": 200,
"bodyFileName": "hall-of-famers.json"
}
}
5 - Spin up docker
In your docker root directory:
docker-compose up
Check the logs of your wiremock image:
docker logs [server-prefix]-wiremock-wwe-server-1
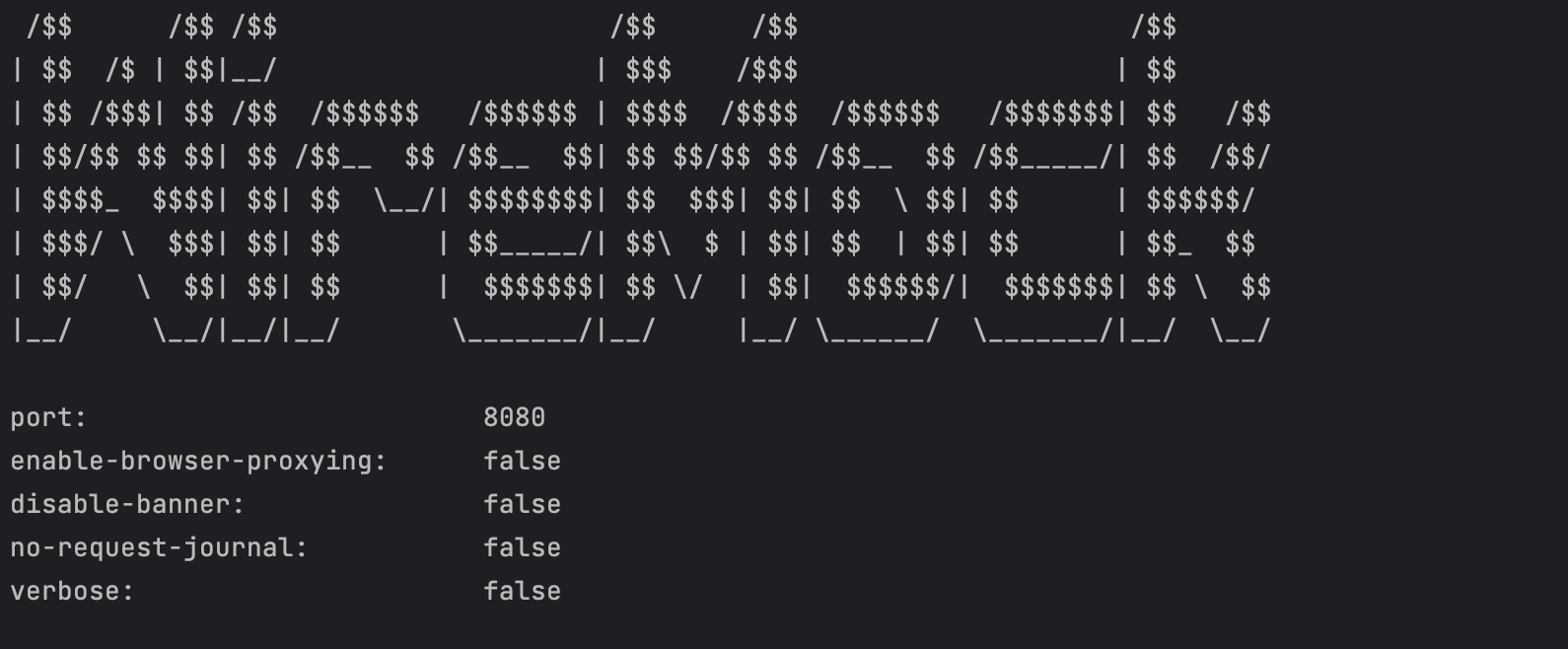
6 - Test API call to our mock
This is a good spot to stop and test the API call
curl -GET -H "Content-type: application/json"
'http://localhost:3001/api/v1/hall-of-fame'
Lo and behold, we get a response!
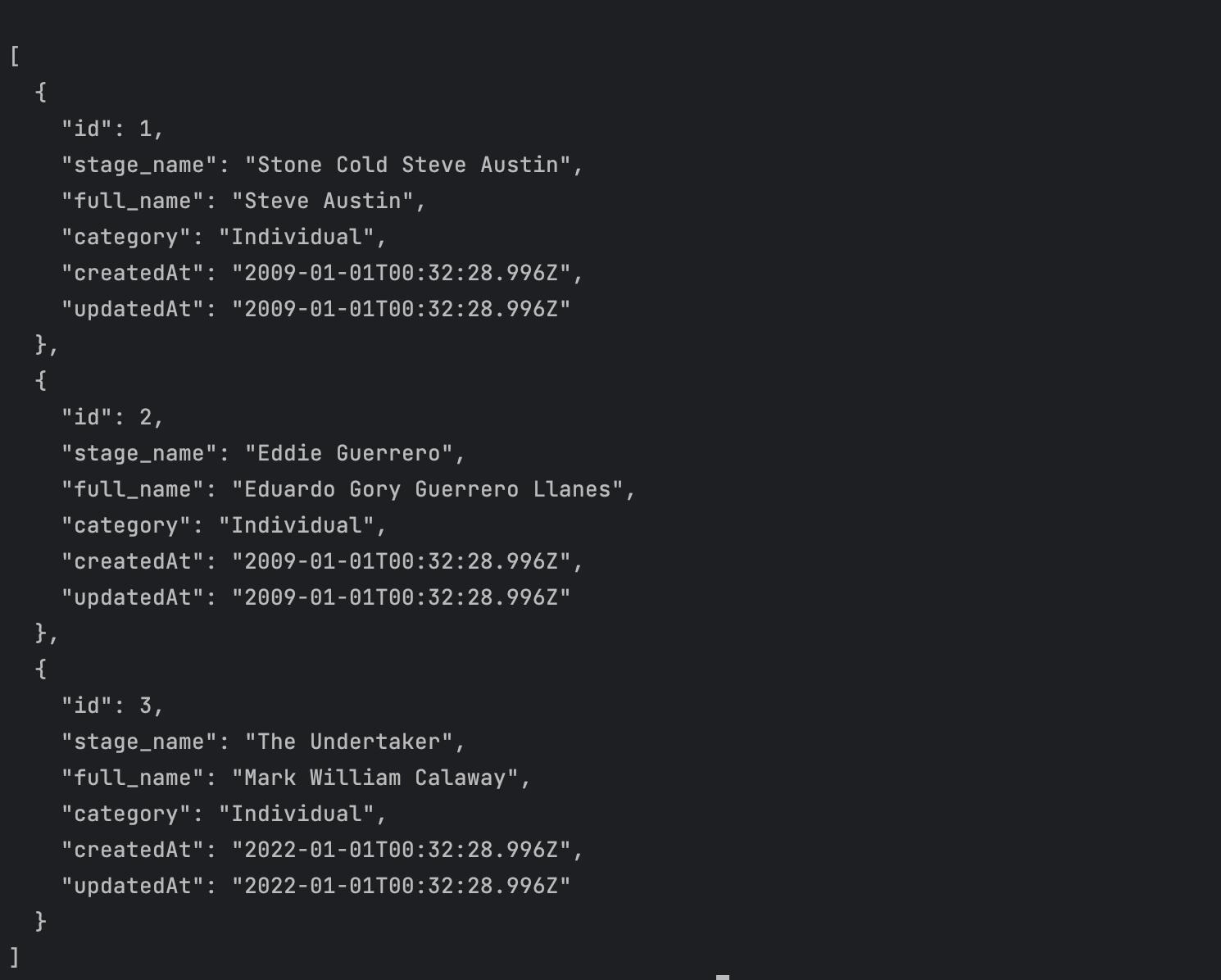
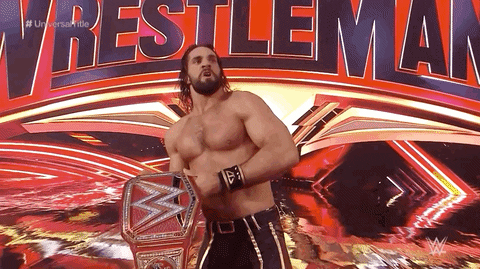
Down to the wire
As you can see, The API calls have… spoiler: a mediocre joke ahead - No Way Out 🥁 (former WWE wrestling event). I find it very handy when I have a small change and I don’t want to waste time on building and running multiple services. By using simple JSON files I’m able to mock the external APIs response and continue to work on my own pace. Another reason I find mocking useful is having a more stable testing infrastructure when the external API can be flaky, limited, expensive or just slow.
Pros
- You can run faster in your testing or development without having to run a complete local setup.
- You save calls to external services which comes in handy to avoid hitting API rate limit.
Cons
- You’ll need to plan your mock API calls. Mapping is not dynamic, so make sure you align your request payload with the data. However, you can use regex for matching different query params.
- You’ll have to maintain your data with the expected response of the external API calls.
🔔 Ding Ding Ding
Ok winners, I hope this tutorial helped you out! You can do a lot more with Wiremock so use this baseline to enrich the requests with headers, query params, body payload and more. You can even create stub mappings from requests by recording it. For additional documentation head over to Wiremock docs .
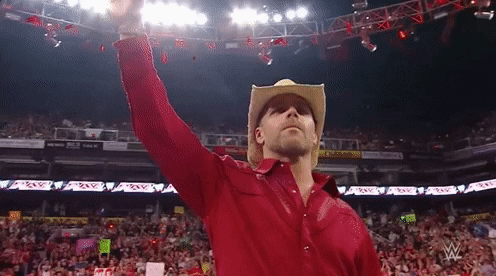